If you are looking to learn how to create CRUD application in PHP then you are in the right place. In a web application, 4 operations are mainly performed, Create, Read, Update and Delete. These are called CRUD operations in short.
- Create – This is to save the data to the database.
- Read – This is the operation to read or display the saved data from the database.
- Update – This is to update the existing records in a database.
- Delete – Last but not least, this is used to perform delete operation in the database.
Each operation has its own query syntax to perform its task. Let’s take a look at each query and we will understand its concepts.
Check this also how to submit form data using AJAX in PHP to learn to perform a single CRUD operation that is to create without page refresh. You check this demo also.
Ultimately we perform CRUD operation in any complex web application or website by different techniques and methods.
Today, I am very happy to share with you CRUD application in PHP using jQuery AJAX from scratch. But today I will show you a very simple way to CRUD using a bootstrap popup model.
How to do that?
In this tutorial, I will use a bootstrap popup model in which I will place a registration form asking to fill name, email, and contact.
After that, I will fetch the records from the database and display them without page refresh that’s why we are using AJAX.
Resources to perform CRUD operations
- It is mandatory to use jQuery library to use AJAX functionality.
- Here I will use a popup model on the click of the add button so that we can do this without a page refresh, That’s why we need bootstrap also. This will be good for styling purposes too.
- That’s enough!
Lets first see the files and folder structure we are going to create for CRUD application in PHP using jQuery AJAX.
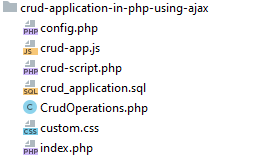
Let’s understand the task of these files. Each file has its own task.
- config.php is the connection file to the database.
- crud-app.js is a javaScript file responsible to make AJAX call to accomplish not to page refresh.
- crud-script.php has the logics of CRUD means to create, read, delete and update.
- CrudOprations.php is a PHP class that has user-defined functions. Since we are going to use the OOPs methodology.
- custom.css is CSS file to just to style something nothing else.
- finally, index.php is the main file on which everything is to be performed.
crud_applications.sql is just an SQL file which is a database table. The table has this structure and you to create this in your database.

Don’t worry, I will provide the SQL file, Source code link is at the end of this post. Please include these CDN in the head tag of your index.php file.
<link rel="shortcut icon" href="https://demo.codingbirdsonline.com/website/img/coding-birds-online/coding-birds-online-favicon.png"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"/>
and these JavaScript files to the footer means above the body tag in the index.php.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script src="crud-app.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
complete index.php file
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>CRUD Application in PHP using AJAX - Coding Birds Online</title> <link rel="shortcut icon" href="https://demo.codingbirdsonline.com/website/img/coding-birds-online/coding-birds-online-favicon.png"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"/> <link rel="stylesheet" href="custom.css"> </head> <body> <div style="margin-top: 10px;"><img width="100" src="https://demo.codingbirdsonline.com/website/img/coding-birds-online/coding-birds-online-favicon.png" class="center-block"/></div> <div class="container"> <h3>Delete multiple records from database using PHP </h3> <a href="javaScript:void(0);" data-toggle="modal" data-target="#myModal" class="btn btn-primary pull-right bottom-gap">Add New <i class="fa fa-plus" aria-hidden="true"></i></a> <table class="table table-bordered"> <thead id="thead" style="background-color:#135361"> <tr> <th>Sr.No</th> <th>Name</th> <th>Email</th> <th>Contact</th> <th>Action</th> </tr> </thead> <tbody id="crudData"></tbody> </table> </div> <div class="modal fade" id="myModal" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal">×</button> <h4 class="modal-title">CRUD Application Form</h4> </div> <div class="modal-body"> <form id="crudAppForm"> <div class="row"> <div class="col-md-4"> <div class="form-group"> <label for="name">Name <span class="field-required">*</span></label> <input type="text" name="name" id="name" placeholder="Name" class="form-control"> </div> </div> <div class="col-md-4"> <div class="form-group"> <label for="email">Email <span class="field-required">*</span></label> <input type="text" name="email" id="email" placeholder="Email" class="form-control"> </div> </div> <div class="col-md-4"> <div class="form-group"> <label for="contact">Contact <span class="field-required">*</span></label> <input type="text" name="contact" id="contact" placeholder="Contact" class="form-control"> </div> </div> </div> <div class="row"> <div class="col-md-4"> <input type="hidden" name="editId" id="editId" value="" /> <button type="submit" name="submitBtn" id="submitBtn" class="btn btn-primary"><i class="fa fa-spinner fa-spin" id="spinnerLoader" ></i> <span id="buttonLabel">Save</span> </button> </div> </div> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> </div> </div> </div> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script src="crud-app.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </body> </html>
crud-app.js file
$( document ).ready(function() { getAllData(); console.log( "ready!" ); }); $("form#crudAppForm").on("submit",function (e) { e.preventDefault(); var name = $("#name").val(); var email = $("#email").val(); var contact = $("#contact").val(); var editId = $("#editId").val(); if (name == ""){ alert("Please enter name"); $("#name").focus(); }else if (email == "") { alert("Please enter email"); $("#email").focus(); }else if (contact == "") { alert("Please enter contact"); $("#contact").focus(); }else{ $("#buttonLabel").html("Saving..."); $("#spinnerLoader").show('fast'); $.post("crud-script.php",{ crudOperation:"saveData", name:name, email:email, contact:contact, editId:editId, },function (response) { if (response == "saved") { $("#buttonLabel").html("Save"); $("#spinnerLoader").hide('fast'); $("#myModal").modal('hide'); $("form#crudAppForm").each(function () { this.reset(); }); getAllData(); } }); } }); function getAllData() { $.post("crud-script.php",{crudOperation:"getData"},function (allData) { $("#crudData").html(allData); }); } function editData(editId,name,email,contact) { $("#editId").val(editId); $("#name").val(name); $("#email").val(email); $("#contact").val(contact); $("#myModal").modal('show'); } function deleteData(deleteId) { if(confirm("Are you sure to delete this ?")){ $('#deleteSpinner'+deleteId).show('fast'); $.post("crud-script.php",{crudOperation:"deleteData",deleteId:deleteId},function (response) { if (response == "deleted") { $('#deleteSpinner'+deleteId).hide('fast'); getAllData(); } }); } }
crud-script.php file
<?php include "config.php"; include_once "CrudOperations.php"; $crudObj = new CrudOperations(); if ($_POST['crudOperation'] == "saveData") { $name = $_POST['name']; $email = $_POST['email']; $contact = $_POST['contact']; $editId = $_POST['editId']; $save = $crudObj->saveData($connection,$name,$email,$contact,$editId); if ($save){ echo "saved"; } } if ($_POST['crudOperation'] == "getData") { $sr = 1; $tableData = ''; $allData = $crudObj->getAllData($connection); if ($allData->num_rows>0) { while ($row = $allData->fetch_object()) { $tableData .= ' <tr> <td>'.$sr.'</td> <td>'.$row->name.'</td> <td>'.$row->email.'</td> <td>'.$row->contact.'</td> <td><a href="javaScript:void(0)" onclick="editData(\''.$row->id.'\',\''.$row->name.'\',\''.$row->email.'\',\''.$row->contact.'\');" class="btn btn-success btn-sm">Edit <i class="fa fa-pencil-square-o"></i></a> <a href="javaScript:void(0)" onclick="deleteData(\''.$row->id.'\');" class="btn btn-danger btn-sm">Delete <i class="fa fa-trash-o"></i></a> <i class="fa fa-spinner fa-spin" id="deleteSpinner'.$row->id.'" style="color: #ff195a;display: none"></i></td> </tr>'; $sr++; } } echo $tableData; } if ($_POST['crudOperation'] == "deleteData"){ $deleteId = $_POST['deleteId']; $delete = $crudObj->deleteData($connection,$deleteId); if ($delete){ echo "deleted"; } }
config.php file
<?php $connection = new mysqli("localhost","root","","codingbirds"); if (! $connection){ die("Error in connection".$connection->connect_error); }
CrudOperations.php file
<?php class CrudOperations { public function saveData($connection,$name,$email,$contact,$editId) { if ($editId == "") { $query = "INSERT INTO crud_application SET name='$name',email='$email',contact='$contact'"; }else{ $query = "UPDATE crud_application SET name='$name',email='$email',contact='$contact' WHERE id='$editId'"; } $result = $connection->query($query) or die("Error in saving data".$connection->error); return $result; } public function getAllData($connection) { $query = "SELECT * FROM crud_application"; $result = $connection->query($query) or die("Error in getting data".$connection->error); return $result; } public function deleteData($connection,$deleteId){ $query = "DELETE FROM crud_application WHERE id='$deleteId'"; $result = $connection->query($query) or die("Error in deleting data".$connection->error); return $result; } }
custom.css
#thead>tr>th{ color: white; } .center-block { display: block; margin-left: auto; margin-right: auto; } .bottom-gap{ margin-bottom: 10px; } .field-required,.delete-spinner{ color: red; } #spinnerLoader{ display: none; }
Run the Code!
Now if you run the code, if there are not any errors you will get something like this.

When you will submit this form you will the complete final output like this. And if you click on the Add New+ button this popup form will have appeared.

If you submit this form filling the asked values then the record will be displayed. And to inform you, everything will be on the same page without any reload. Again if you click on the edit button then the same bootstrap popup will appear with the values which you filled previously.
If you update the values then it will call ajax request, update them to the database and display again to the table.
So if you want to delete you can do it also. It will ask you “Are you sure to delete this ?”. If you confirm this then it will be deleted.
Source Code & Demo
You can download the full 100% working source code from here. You check this demo also.
Conclusion
I hope you learned explained above, If you have any suggestions, are appreciated. And if you have any errors comment here. You can download the full 100% working source code from here.
Ok, Thanks for reading this article, see you in the next post.
Happy Coding 🙂