Hi, are you looking for the code in which you want to delete the multiple records using a checkbox? In this tutorial, you will learn to delete multiple records in PHP using the checkbox or selected checkbox.
In the previous tutorial, you learned about how to delete a single record. Here you learn about to delete the multiple records by selected checkbox with validation to select at least one checkbox. Here are the files and folders to delete multiple records in PHP. Check this demo.
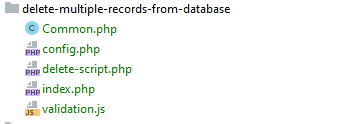
So without wasting our time, let’s get started to create our files.
index.php
<!DOCTYPE html>
<html lang="en">
<head>
<title>Delete records from database using PHP - Coding Birds Online</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="validation.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon">
<style>
#thead>tr>th{ color: white; }
</style>
</head>
<body>
<div style="margin-top: 20px;padding-bottom: 20px;">
<center>
<img width="100" src="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png"/>
<h3>Delete multiple records from database using PHP </h3>
</center>
</div>
<div class="container">
<form action="delete-script.php" method="post">
<table class="table table-bordered">
<thead id="thead" style="background-color:#1573ff">
<tr>
<th>Sr.No</th>
<th>Name</th>
<th>Email</th>
<th>Contact</th>
<th>Department</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<?php
include "config.php";
include_once "Common.php";
$common = new Common();
$records = $common->getAllRecords($connection);
if ($records->num_rows>0){
$sr = 1;
while ($record = $records->fetch_object()) {
$recordId = $record->id;
$name = $record->name;
$email = $record->email;
$contact = $record->contact;
$department = $record->department;?>
<tr>
<td><?php echo $sr;?></td>
<td><?php echo $name;?></td>
<td><?php echo $email;?></td>
<td><?php echo $contact;?></td>
<td><?php echo $department;?></td>
<td><input type="checkbox" name="recordsCheckBox[]" id="recordsCheckBox" value="<?php echo $recordId;?>"></td>
</tr>
<?php
$sr++;
}
}
?>
</tbody>
</table>
<input type="submit" name="deleteBtn" id="deleteBtn" class="btn btn-success" onclick="return validateForm();" value="Delete Records" style="float: right"/>
</form>
</div>
</body>
</html>
This index.php file includes config.php and Common.php. These files are database connections and PHP class file. Let’s create these files.
config.php
<?php
$hostName = "localhost"; // host name
$username = "root"; // database username
$password = ""; // database password
$databaseName = "codingbirds"; // database name
$connection = new mysqli($hostName,$username,$password,$databaseName);
if (!$connection) {
die("Error in database connection". $connection->connect_error);
}
?>
Common.php
<?php
class Common
{
public function getAllRecords($connection) {
$query = "SELECT * FROM bird_delete_records";
$result = $connection->query($query) or die("Error in query1".$connection->error);
return $result;
}
public function deleteRecordById($connection,$id) {
$query = "DELETE FROM bird_delete_records WHERE id='$id'";
$result = $connection->query($query) or die("Error in query".$connection->error);
return $result;
}
}
validation.js
function validateForm() {
var count_checked = $("[name='recordsCheckBox[]']:checked").length;
if (count_checked == 0) {
alert("Please check at least one checkbox");
return false;
}else{
return true;
}
}
delete-script.php
<?php
include "config.php";
include_once "Common.php";
if (isset($_POST['deleteBtn'])){
$recordIds = $_POST['recordsCheckBox'];
$common = new Common();
foreach ( $recordIds as $id ) {
$delete = $common->deleteRecordById($connection,$id);
}
if ($delete) {
echo '<script>alert("Record deleted successfully !")</script>';
echo '<script>window.location.href="index.php";</script>';
}
}
After running this code you will get output like this.
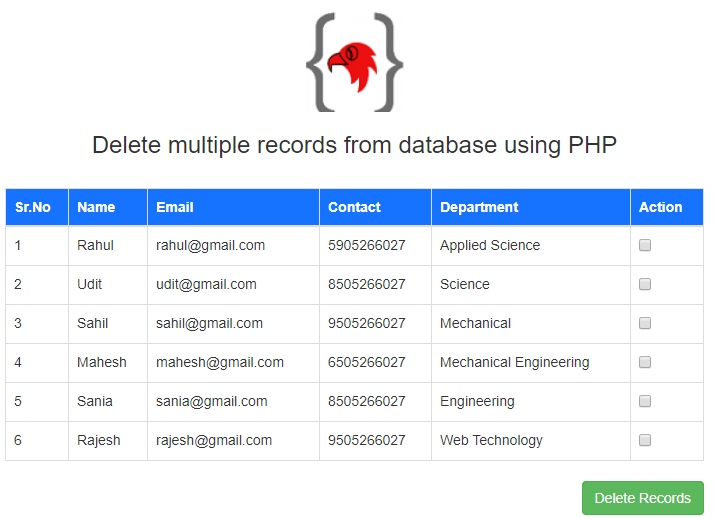
So this is the output when you run the code. Now if you press the delete button when you get a prompt alert that, select at least one checkbox.
After selecting and pressing the delete button the records will be deleted that you have selected before. I hope you understood the concept and the code.
You can download the full working source code from here so that there is no error in your code. You can also watch this video uploaded a year ago of mine, telling the same thing but in procedural style.
Happy Coding 🙂