Hey! Are you looking to create a country-state-city select dropdown? Here I will give you a quick guide with source code so that you can implement it in your application in 0 errors. Check this demo.
I am Ankit and today I am gonna tell you about how you can code this select Dropdown in an easy way.
This dropdown is a dependency dropdown between the country, state, city. When one dropdown value depends upon another dropdown value then it is called dependent or dependency dropdown.
About Country-state-city dropdown
In this tutorial, we will create an HTML form with 3 select dropdowns. Which is Country, State, City respectively? To get states first I will select a country from the dropdown, and after that to get city I will select a state. This is how dependent dropdown works together. Don’t worry I will explain how to make them dependent on each other.
What are the requirements?
First of all, you need to have a number of countries in a country table in your database with a country id that is unique.
Country Table

State Table
Then after, you need to have a states table in your database with entries of various states and a column on country id, so that you can identify the state name by country id.

City Table
Then finally you need to have a city table contains the number of cities with an id of states accordingly.
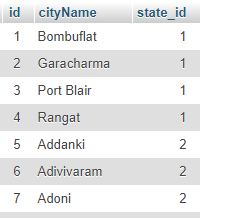
What is Logic ??
First I will fetch all the countries from the country table and display them to the first select dropdown, Rest two select dropdowns are empty.
Now I will call the onchange function of javaScript and make an AJAX request by taking the country id.
Then based on the country id, I will query the database and select the number of states which have this country id corresponding to the rows of the state table.
Now states dropdown has come. It is time to get cities based on state selection. Again I will call the function on the selection of state and make an AJAX request by taking the state id and query to the database as above.
Don’t worry I will give this data of SQL files like country, state, city table
Let’s see the folder structure.
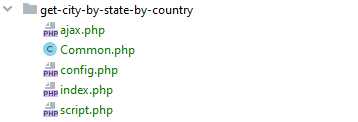
Step by step process
- First I will create an index.php file which is our mail file. I will create 3 select dropdowns in HTML of country, state, and city.
- index.php file has the form tag with the action of script.php. Script.php is to simply print after submitting button.
- Now I will create an ajax.php file, which is the logic file that receives the id of states and country depending upon AJAX request.
- config.php is the connection file to the database.
- Common.php is the PHP class file, which is responsible to query to the database.
Now its time to create these files. Let’s do it.
index.php
<!DOCTYPE html>
<html lang="en">
<head>
<title>Upload Excel(CSV) file with PHP - CodingBirdsOnline.com</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon">
<style>
input[type=text], select {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
input[type=submit] {
width: 100%;
background-color: #4CAF50;
color: white;
padding: 10px;
margin: 8px 0;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: #45a049;
}
#box {
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
margin: 0px 200px 0px 200px;
}
</style>
</head>
<body>
<div class="jumbotron text-center">
<h1>Get City by State by Country</h1>
<p>https://codingbirdsonline.com</p>
</div>
<div id="box">
<form action="script.php" method="post">
<?php
include "config.php";
include_once "Common.php";
$common = new Common();
$countries = $common->getCountry($connection);
?>
<label>Country <span style="color:red">*</span></label>
<select name="country" id="countryId" class="form-control" onchange="getStateByCountry();">
<option value="">Country</option>
<?php
if ($countries->num_rows > 0 ){
while ($country = $countries->fetch_object()) {
$countryName = $country->name; ?>
<option value="<?php echo $country->id; ?>"><?php echo $countryName;?></option>
<?php }
}
?>
</select>
<label>State <span style="color:red">*</span></label>
<select class="form-control" name="state" id="stateId" onchange="getCityByState();" >
<option value="">State</option>
</select>
<label>City <span style="color:red">*</span></label>
<select class="form-control" name="city" id="cityDiv">
<option value="">City</option>
</select>
<input type="submit" value="Submit">
</form>
</div>
<script>
function getStateByCountry() {
var countryId = $("#countryId").val();
$.post("ajax.php",{getStateByCountry:'getStateByCountry',countryId:countryId},function (response) {
var data = response.split('^');
$("#stateId").html(data[1]);
});
}
function getCityByState() {
var stateId = $("#stateId").val();
$.post("ajax.php",{getCityByState:'getCityByState',stateId:stateId},function (response) {
var data = response.split('^');
$("#cityDiv").html(data[1]);
});
}
</script>
</body>
</html>
ajax.php
<?php
include "config.php";
include_once "Common.php";
if (isset($_POST['getStateByCountry']) == "getStateByCountry") {
$countryId = $_POST['countryId'];
$common = new Common();
$states = $common->getStateByCountry($connection,$countryId);
$stateData = '<option value="">State</option>';
if ($states->num_rows>0){
while ($state = $states->fetch_object()) {
$stateData .= '<option value="'.$state->id.'">'.$state->statename.'</option>';
}
}
echo "test^".$stateData;
}
if (isset($_POST['getCityByState']) == "getCityByState") {
$stateId = $_POST['stateId'];
$common = new Common();
$cities = $common->getCityByState($connection,$stateId);
$cityData = '<option value="">City</option>';
if ($cities->num_rows>0){
while ($city = $cities->fetch_object()) {
$cityData .= '<option value="'.$city->id.'">'.$city->cityName.'</option>';
}
}
echo "test^".$cityData;
}
Common.php
<?php
/**
* Created by PhpStorm.
* User: Ankit
* Date: 11/29/2018
* Time: 7:50 PM
*/
class Common
{
public function getCountry($connection)
{
$mainQuery = "SELECT * FROM bird_countries";
$result1 = $connection->query($mainQuery) or die("Error in main Query".$connection->error);
return $result1;
}
public function getStateByCountry($connection,$countryId){
$query = "SELECT * FROM bird_states WHERE countryId='$countryId'";
$result = $connection->query($query) or die("Error in Query".$connection->error);
return $result;
}
public function getCityByState($connection,$stateId){
$query = "SELECT * FROM bird_cities WHERE state_id='$stateId'";
$result = $connection->query($query) or die("Error in Query".$connection->error);
return $result;
}
}
script.php
<?php
if (isset($_POST['submit'])) {
$country = $_POST['country'];
$state = $_POST['state'];
$city = $_POST['city'];
echo 'Country is: '. $country; echo '<br/>';
echo 'State is : '. $state; echo '<br/>';
echo 'City is : '. $city; echo '<br/>';
}
config.php
<?php
$connection = new mysqli("localhost","root","","codingbirds");
if (! $connection){
die("Error in connection".$connection->connect_error);
}
Now after creating these files to your editor, importing tables which I will provide and making a connection to your database with config.php file, Its time to test it. When you run it you will get output like below.

Now as you see out in the browser, if you select a country, the states will appear according to that country. If you select the state then city as simple as that. You can also put a loader indicator while data is loading on the selected country. Learn how to create loading spinner indicator in jQuery ajax.
Source Code & Demo
So you enjoyed this tutorial and learned how to code this in easy ways. If you have any problem you can download the full working source code here. Check this demo.
Conclusion
In this tutorial, you learned about how to make country-state-city dependent dropdown or cascading dropdown. I hope you have enjoyed this tutorial. If there is anything for improvement, let me know I will be happy. If you face any problem with doing this, you can comment I will help.
30 Comments
Great info here! Thanks for this article. I have no idea about coding!
Plz help selected items after submit output data display in table form
I was not very much aware of the coding. Very useful article. I am very poor in this area, am learning slowly.
I was searching for this code. You have really solved my problem. Thanks
Thank you for sharing this is such a great resource!
This is broken down very well! Thanks for the info!
It is so complicated, I don’t really understand
Great info it would help a lot I’m still learning in coding Lol thanks for this
Thanks for sharing this great information! I’m still new to coding and I found this to be so helpful.
I didn’t know you could do such a thing in PHP! Thanks for the amazing information!
Oh wow, this would have been tricky to write. Thanks for doing the hard work and sharing your codes for everyone to use!
Coding still gives me a headache even though i have been practicing . Thank you for sharing.
Thanks for your appreciation, It matters a lot.
Thank you for sharing your expertise on this technical matter. This is a very detailed tutorial that I will have to refer back to as needed.
Very helpful article. Thank you for sharing.
Dear Ankit,
Thank for sharing this useful information….
greatness in this blog is things are very well described and site structure is so easy to understand.
thank you so much…
keep doing this.
Very useful article, much appreciated Ankit!
Plz help selected items after submit output data display in table form
Selected mens after submit data how to display in output, plz help
When we select country as American samoa and state as Sawins Island, we are not observing any list for the cities. This will major problem we these fields are required.
This is because there are no entries for “Sawins Island”, If you want to get those as cities, just check the id of “Sawins Island” in the state table, let say 60. Now in the City table, insert the city name and given a state_id to be 60.
Good Job!
Countries show but states and cities don’t show.
Any help?
Nice tutorials, it works! but when It inserts in inserts values digits instead of the text! Can you help me out!
Please check the type of column in the database. It should be varchar to save text.
Hi I was looking at your really good example (How to make country state city dropdown in PHP)
I am not a developer like yourself, but I need to create a similar project like your
I am getting this error and I do not understand what it means
Fatal error: Uncaught Error: Call to undefined method Common::getCountries() in C:\xampp\htdocs\pc\room\index.php:55 Stack trace: #0 {main} thrown in C:\xampp\htdocs\pc\room\index.php on line 55
Can you please kindly help me?
I’m really loving the theme/design of your
site. Do you ever run into any web browser compatibility issues?
A handful of my blog readers have complained about my site not operating correctly in Explorer but looks great
in Firefox. Do you have any suggestions to help fix this problem?
Touche. Great arguments. Keep up the great spirit.
In India, there are a total of 36 states but in the bird_states there are showing a total of 41 states, and related to the United Kingdom the states are not showing as expected.
This is just an example, I just pull SQL data from Google, However can customize as per your needs.