Hellowww friends! after a long time I am back again with another post. Today I have come with stripe Payment Gateway Tutorial. So let’s start the post. Let’s talk about stripe payment gateway integration.
Stripe is one of the most used payment gateway used across the world. It is a cloud payment gateway platform that helps to accept and manage online transactions anywhere in the world. It provides complete solutions to process online payments and also offers great features like a custom UI toolkit, embeddable checkout, consolidated reports, and much more.
To start payment gateway integration in PHP you need to have a merchant/owner account in stripe means if you have a business or any e-commerce website and want to accept online payment then you need to have an owner account. You can create and upload other verifications details like bank account and identity details. I hope you have completed these steps and just looking for code and procedure to integrate. So let’s move to the next step. Check this demo.
Get your API keys
Login to your stripe dashboard, go to the right top corner under the profile dropdown you will see the developer link. Find the secret key and copy keep it somewhere we are going to use it.
Project & Code Setup
Now we need an official Stripe PHP library. If you composer then it’s fine otherwise we need to install the composer. Composer is a dependency manager tool. Click here to download it from the composer website and install it. It is very simple to install. Now create a project folder in your xampp/wampp project directory.
After you have created the project folder, open CMD inside the project directory and run the below command.
Stripe payment gateway integration
Install Stripe PHP Library
composer require stripe/stripe-php
After your command completes it will create a vendor folder, composer.json & composer.lock files. We don’t need to touch these files. Now rest of the files we need to create our project directory.

index.php is the home page which is the simple event registration & fee payment form. This is we are going to have a number of registrations and accept fees via stripe. Here is the code of this file.
Database Setup
To keep the number of registrations and payment status we need to create a database. In this case database name is “codingbirds” and the table is called “bird_stripe_payments“
After creating the database go to SQL and paste this code and run to create the table.
-- -- Table structure for table `bird_stripe_payments` -- CREATE TABLE `bird_stripe_payments` ( `id` int(10) NOT NULL, `name` varchar(255) NOT NULL, `email` varchar(255) NOT NULL, `contact` varchar(12) NOT NULL, `amount` decimal(10,2) NOT NULL, `payment_status` enum('Pending','Completed') NOT NULL DEFAULT 'Pending', `payment_intent` text DEFAULT NULL, `created_at` datetime NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
Code & Files Setup
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.bundle.min.js"></script> <title>How to integrate stripe payment gateway in php - Coding Birds Online</title> <style> .required { color: red; font-weight: bold }</style> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-9 col-md-7 col-lg-5 mx-auto"> <div class="card card-signin my-5"> <div class="card-body"> <center> <img src="https://codingbirdsonline.com/wp-content/uploads/2020/01/cropped-coding-birds-online-favicon-180x180.png" width="50"/> </center> <br/> <h5 class="card-title text-center">Join This College Event</h5> <form action="checkout.php" method="post"> <div class="form-group"> <label for="name">Name <span class="required">*</span></label> <input type="text" name="name" class="form-control" placeholder="Name" required/> </div> <div class="form-group"> <label for="email">Email <span class="required">*</span></label> <input type="email" name="email" id="email" class="form-control" placeholder="Email" required/> </div> <div class="form-group"> <label for="contact">Contact <span class="required">*</span></label> <input type="text" name="contact" id="contact" class="form-control" placeholder="Contact" maxlength="10" required/> </div> <div class="form-group"> <label for="amount">Fee Amount <span class="required">*</span></label> <input type="text" name="amount" id="amount" class="form-control" placeholder="Amount" value="100" readonly required/> </div> <button type="submit" name="payNowBtn" class="btn btn-lg btn-primary btn-block">Pay Now <span class="fa fa-angle-right"></span></button> </form> </div> </div> </div> </div> </div> </body> </html>
In the index.php file, we have the form action of checkout.php. This file contains the main logic of creating products and prices and creates payment links and redirects. Here is the code.
<?php session_start(); require_once 'Database.php'; require_once "StripeHelper.php"; require_once "User.php"; /** * APP url/base url */ $appUrl = "http://localhost/projects/codingbirds_/integrate-stripe-payment-gateway-in-php/"; $productPrice = 100; // calculate as per logic // $database = new Database(); /** * When registration form submitted */ if (isset($_POST['payNowBtn'])){ $user = new User(); $data = $_POST; $data['amount'] = $productPrice; $user = $user->registerUser($database->connection, $_POST); $_SESSION['user_id'] = $database->connection->insert_id; } $stripeHelper = new StripeHelper(); $stripe = $stripeHelper->stripeClient; /** * Create product */ $product = $stripeHelper->createProducts(); /** * Create price for product */ $price = $stripeHelper->createProductPrice($product, $productPrice); /** * create checkout session and payment link */ $stripeSession = $stripe->checkout->sessions->create( array( 'success_url' => $appUrl . 'success.php?session_id={CHECKOUT_SESSION_ID}', 'cancel_url' => $appUrl . 'failed.php', 'payment_method_types' => array('card'), 'mode' => 'payment', 'line_items' => array( array( 'price' => $price->id, 'quantity' => 1, ) ) ) ); header("Location: " . $stripeSession->url);
In this file, we have included other helper class files Database.php, StripeHelper.php & User.php. Here is the code of these files.
Database.php
<?php include_once "constants.php"; class Database { /** * @var mysqli */ public $connection; public function __construct() { $this->connection = new mysqli(DB_HOST,DB_USERNAME,DB_PASSWORD,DB_NAME); if (!$this->connection) { die("Error in database connection". $this->connection->connect_error); } } }
constants.php file code is
<?php /** * Database configuration */ define('DB_HOST', 'localhost'); define('DB_USERNAME', 'root'); define('DB_PASSWORD', ''); define('DB_NAME', 'codingbirds'); /** * Stripe API configuration */ define('STRIPE_API_SECRET_KEY', 'xxxxxxxxxxx');
StripeHelper.php
<?php require 'vendor/autoload.php'; include_once "constants.php"; class StripeHelper { /** * @var \Stripe\StripeClient */ public $stripeClient; public function __construct() { $this->stripeClient = new \Stripe\StripeClient(STRIPE_API_SECRET_KEY); } /** * Create product * @return \Stripe\Product * @throws \Stripe\Exception\ApiErrorException */ public function createProducts() { return $this->stripeClient->products->create(array( 'name' => 'Course 1', 'description' => 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.', )); } /** * Create price * @param $product * @param $productPrice * @return \Stripe\Price * @throws \Stripe\Exception\ApiErrorException */ public function createProductPrice($product, $productPrice) { return $this->stripeClient->prices->create(array( 'unit_amount' => $productPrice * 100, 'currency' => 'INR', 'product' => $product->id, )); } /** * Get session detail * @param $sessionId * @return \Stripe\Checkout\Session * @throws \Stripe\Exception\ApiErrorException */ public function getSession($sessionId) { return $this->stripeClient->checkout->sessions->retrieve($sessionId); } }
success.php
<?php session_start(); require_once "Database.php"; require_once "StripeHelper.php"; require_once "User.php"; $stripeHelper = new StripeHelper(); if(isset($_SESSION['user_id'])){ $user = new User(); $database = new Database(); $sessionId = $_GET['session_id']; $userId = $_SESSION['user_id']; $checkoutSession = $stripeHelper->getSession($sessionId); $user->updatePaymentStatus($database->connection, $checkoutSession->payment_intent, $userId); }else{ header("Location: index.php"); } //echo '<pre>'; ///print_r($checkoutSession); ?> <!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.bundle.min.js"></script> <title>Success | How to integrate stripe payment gateway in php - Coding Birds Online</title> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-9 col-md-7 col-lg-5 mx-auto"> <div class="card card-signin my-5"> <div class="card-body" style="outline: 2px solid #23e323"> <h5 class="card-title text-center">Payment success</h5> <p>Transaction ID: <?php echo $checkoutSession->payment_intent; ?> </p> </div> </div> </div> </div> </div> </body> </html>
failed.php
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.bundle.min.js"></script> <title>Failed | How to integrate stripe payment gateway in php - Coding Birds Online</title> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-9 col-md-7 col-lg-5 mx-auto"> <div class="card card-signin my-5"> <div class="card-body" style="outline: 2px solid red"> <h5 class="card-title text-center">Payment failed</h5> </div> </div> <a href="./index.php" class="btn btn-primary">Try again </a> </div> </div> </div> </body> </html>
User.php
<?php class User { /** * Register the user * @param $connection * @param $data * @return mixed */ public function registerUser($connection, $data) { $date = date('Y-m-d H:i:s'); $query = "INSERT INTO bird_stripe_payments SET name ='{$data['name']}',email='{$data['email']}', contact='{$data['contact']}',amount='{$data['amount']}', created_at='{$date}' "; $result = $connection->query($query) or die("Error in query".$connection->error); return $result; } /** * Update the payment status * @param $connection * @param $txnId * @param $userId * @return mixed */ public function updatePaymentStatus($connection, $txnId, $userId) { $query = "UPDATE bird_stripe_payments SET payment_status='Completed', payment_intent='$txnId' WHERE id='$userId' "; $result = $connection->query($query) or die("Error in query".$connection->error); return $result; } }
After creating these files & code if you run your project then it will look something like this.

After filling in the details click on pay now you will be redirected to the 3rd party stripe page which will handle the payment come back again to our success or fail callback pages.

This is the payment detail page by stripe. This is under test mode you can enter any email name and proceed. Stripe does provide some test details to test the payment. You can try these.
yourtest@gmail.com | 4242 4242 4242 4242 | 02/25 | 123 | Ankit Prajapati |
After successful payment, you will get redirected to the success.php screen
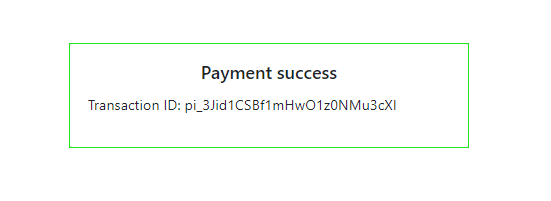
Yes, we can customize the success page according to the needs and requirements.
If payment failed due to any reason or you canceled the payment then you will be redirected to the failed.php file.

Meanwhile, If you notice the checkout.php we create an entry to our database table with user details and payment status pending. After successful payment, we update the payment status to be completed to keep our record updated.

Last but not least you can check all your actual payment transactions and history to your stripe dashboard.
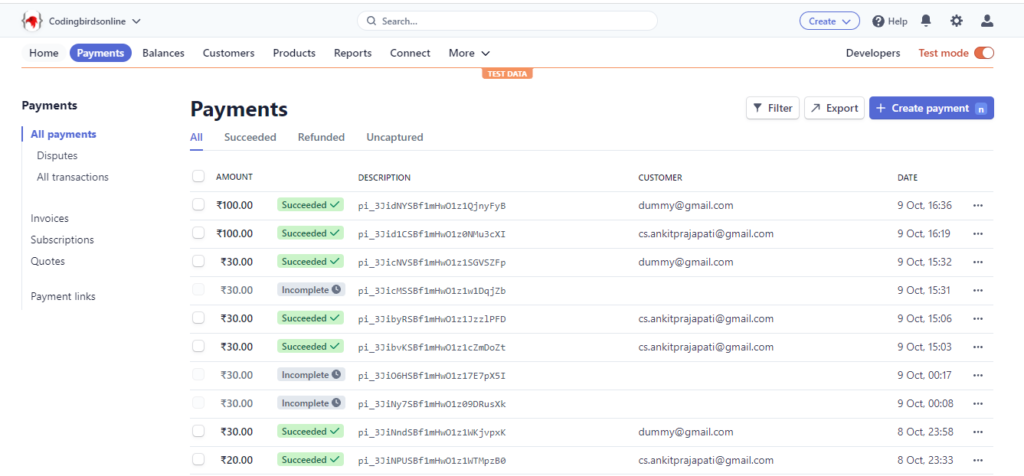
Source Code & Demo
You can download the full 100% working source code from here. You check this demo also.
Conclusion
I hope you learned explained above, If you have any suggestions are appreciated. And if you have any errors comment here, I will help. You can download the full 100% working source code from here.
Ok, Thanks for reading this article, see you in the next post. If this code works for you, please leave a comment so that others can find it useful which gives motivation to me to create such content.
1 Comment
Hi Ankit,
Thanks for the efforts you have put and helping to understand the integration with Stripe. It was very useful to my project. Just have one query around the dynamic quantity. How to write if we want to do with dynamic quantity and multiple products?
Thanks 🙂