Hello everyone, Today in this tutorial I will show you how to create login with Google account using JavaScript OAuth-library in your web application. You can find N number of tutorials on Google authentication but this tutorial is different from them.
This tutorial tells how easy it is to integrate login with Google authentication. In this tutorial, you don’t need to use/download a third partly PHP library provided by Google, unlike other tutorials.
Instead of this you just need to register your app to Google API Console, generate the client Id and secret keys and include a CDN. Don’t worry I will cover each and every step and provide the source code.
Why use Login with Google OAuth library?
Login with Google, JavaScript OAuth library is a quick and powerful way to integrate the login system in the web application. If you do it by using JavaScript, It will enhance the user experience, without page refresh. Google login with JavaScript API allows the user to log in on your website with their Google account.
The best advantage of using the Google JavaScript API library is that the login process can be implemented on a single page without page refresh. Check our demo.
Step by step process to Login with Google account
1- Create a project
To create a project click on this link https://developers.google.com/identity/sign-in/web/sign-in you will get something like this.

Click on the Configure a project button and a popup window appears on the same page with a select dropdown. If you created any project that will be listed, If you didn’t create, click on create project option. Enter a project name and click next. You will get another window as.
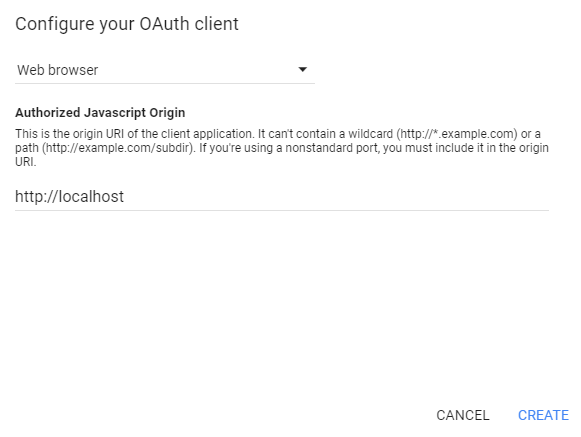
In this first dropdown select “Web browser” and in the Authorized Javascript Origin, enter your URL or domain.
Note:
- If you working on the localhost then the above URL as shown in the picture is correct and will work for the localhost only.
- Ultimately you are going to upload in a live server. In my case, I have a demo on a live server and directory is like https://demo.codingbirdsonline.com/login-with-google-account-using-javascript-OAuth-library/. Means It is in a subdomain.
- Then you need to type this URL https://demo.codingbirdsonline.com. If you want to host your code to subdomain. OR https://codingbirdsonline.com, if want to in root.
- Make sure, you remove forward slash “/” from last.
Click on Create, you will be asked to enter product name or application. This is the name shown during authentication.
Now you will get the Client ID and secret ID.
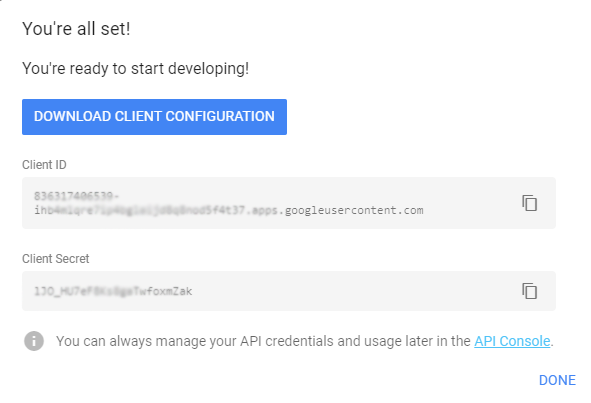
2- Enable API for the project
Open Google Developer Consol, on the top left you will get a dropdown. Select the project that you created in step 1.

Click on the +ENABLE APIS AND SERVICES button and you will be redirected to a page of API. Find Google+ API and enable it.
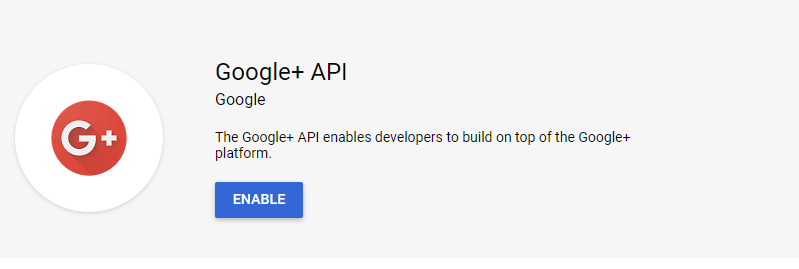
That’s it !!! Now we are just away from our dream. We need some code to configure. In this example, I have a Google Login button. When the user clicks on that, authenticated by his Google account. We save that information provided, for reference. And if he performs the same thing again then we just update our database.
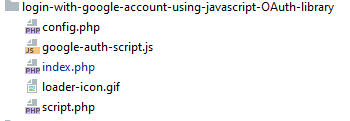
- config.php is the connection file to the database.
- Google-auth-script.js is the JavaScript file to authenticate and saving the data by AJAX.
- index.php is the main file that has the Google Login button.
- loader-icon.gif is the loader icon.
- script.php is the PHP script file that saves data to the database.
Let’s create these files.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://apis.google.com/js/platform.js?onload=renderButton" async defer></script> <meta name="google-signin-client_id" content="your_client_id">
Here, make sure you include Bootstrap and jQuery also. Since we will make AJAX request to save information. Include this above code to the head tag of the index.php file.
Learn more about how to make an AJAX request.
And Include this code to the footer of your index.php file, which means before closing to the body tag.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script src="google-auth-script.js"></script>
index.php: paste this code to the body.
<div class="row"> <div class="col-lg-8 posts-list"> <div class="card card-signin my-5"> <div class="card-body"> <center><img src="https://demo.codingbirdsonline.com/website/img/coding-birds-online/coding-birds-online-favicon.png" width="70"></center> <h5 class="card-title text-center">Login with Google using JavaScript OAuth Library</h5> <center><div id="g-signin2"></div></center> <center><img src="loader-icon.gif" id="loaderIcon" style="display: none"></center> <center> <div class="card" style="width: 22rem;display: none" id="loginDetails"> <img class="" src="" width="100" id="profileLabel" style="margin-top: 20px;"> <div class="card-body"> <div class="card-text" style="text-align: justify"> <table> <tr><td>Name</td><td>:</td><td id="nameLabel"></td></tr> <tr><td>Email</td><td>:</td><td id="emailLabel"></td></tr> <tr><td>Google ID</td><td>:</td><td id="googleIdLabel"></td></tr> </table> </div> <a href="javaScript:void(0)" onclick="signOut();" class="btn btn-danger">Logout</a> </div> </div> </center> </div> </div> </div> </div>
google-auth-script.js.
function renderButton() { gapi.signin2.render('g-signin2', { 'scope': 'profile email', 'width': 250, 'height': 40, 'longtitle': true, 'theme': 'dark', 'onsuccess': onSignIn, 'onfailure': onFailure }); } function onSignIn(googleUser) { var profile = googleUser.getBasicProfile(); console.log('ID: ' + profile.getId()); // Do not send to your backend! Use an ID token instead. console.log('Name: ' + profile.getName()); console.log('Image URL: ' + profile.getImageUrl()); console.log('Email: ' + profile.getEmail()); var googleTockenId = profile.getId(); var name = profile.getName(); var email = profile.getEmail(); var profile = profile.getImageUrl(); $("#loaderIcon").show('fast'); $("#g-signin2").hide('fast'); saveUserData(googleTockenId,name,email,profile); // save data to our database for reference } // Sign-in failure callback function onFailure(error) { alert(error); } // Sign out the user function signOut() { if(confirm("Are you sure to signout?")){ var auth2 = gapi.auth2.getAuthInstance(); auth2.signOut().then(function () { $("#loginDetails").hide(); $("#loaderIcon").hide('fast'); $("#g-signin2").show('fast'); }); auth2.disconnect(); } } function saveUserData(googleTockenId,name,email,profile) { $.post("script.php",{authProvider:"Google",googleTockenId:googleTockenId,name:name,email:email,profile:profile}, function (response) { var data = response.split('^'); if (data[1] == "loggedIn"){ $("#loaderIcon").hide('fast'); $("#g-signin2").hide('fast'); $("#profileLabel").attr('src',profile); $("#nameLabel").html(name); $("#emailLabel").html(email); $("#googleIdLabel").html(googleTockenId); $("#loginDetails").show(); } }); }
By default after page load this function renderButton( ) is called automatically.
script.php
<?php include "config.php"; if ($_POST['authProvider'] == "Google"){ $authProvider = $_POST['authProvider']; $googleTockenId = $_POST['googleTockenId']; $name = $_POST['name']; $email = $_POST['email']; $profile = $_POST['profile']; $now = date('Y-m-d H:i:s'); // Check whether the user data already exist in the database $queryCheck = "SELECT * FROM bird_google_auth WHERE authProvider = '$authProvider' AND googleIdTocken = '$googleTockenId'"; $resultCheck = $connection->query($queryCheck); if ($resultCheck->num_rows>0){ $queryPerform = "UPDATE bird_google_auth SET authProvider = '$authProvider',googleIdTocken = '$googleTockenId',name='$name', email='$email',profile='$profile',modified='$now' WHERE authProvider = '$authProvider' AND googleIdTocken = '$googleTockenId'"; }else{ $queryPerform = "INSERT INTO bird_google_auth SET authProvider = '$authProvider', googleIdTocken = '$googleTockenId',name='$name', email='$email',profile='$profile',created='$now',modified='$now' "; } $finalResultset = $connection->query($queryPerform) or die("Error in query".$connection->error); if($finalResultset){ echo "test^loggedIn"; } }
config.php
<?php $connection = new mysqli("localhost","root","","codingbirds"); if (! $connection){ die("Error in connection".$connection->connect_error); }
3- Run the Code!
When you run the code and if everything is fine. You will get something like this. Don’t worry I will provide the 100% working source code. You only need to change the client ID only.
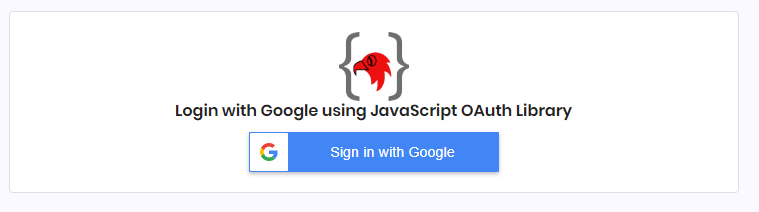


Source Code & Demo
You can download the full 100% working source code from here. You check this demo also.
Conclusion
I hope you learned explained above, If you have any suggestions, are appreciated. And if you have any errors comment here, I will help. You can download the full 100% working source code from here.
Ok, Thanks for reading this article, see you in the next post. If this code works for you, please leave a comment so that others can find code useful.
Happy Coding 🙂