Hi, Are you looking for the PHP script of how to generate QR code in PHP code? then you are right place. Here I will provide the concept, and how to do this. As I think generating QR code with PHP is not complicated nor logical.
You can accomplish this task by using a plugin-play opensource PHP library. So don’t worry. Let’s take a quick introduction to what is the QR code. Check this demo.
What is QR Code?
QR code is the full form of the Quick Response Code 😂. It’s not a joke, I am saying true. You can google it. It is the 2D barcode format code it is used to store text like phone numbers, emails, address, and simple text, or anything want to store, you can.
How to generate QR code in PHP?
I will use a plugin-play opensource PHP library to perform this task. I will create a simple HTML form that accepts text, email, phone or any text for which you want to generate QR code. You can check this demo.
Steps to generate dynamic QR code in PHP
- Create an index.php file, which is a simple HTML form to receive the text.
- Make a generate-qr-script.php, which is a PHP script file to generate QR.
- Create a generate-qr-js.js, which is responsible to generate dynamic QR without page reload.
- And download the QR code library we talked above. Download it from here.
- Finally,create a folder to save QR images. In my terms it is generated-qr.
Files and folder structure
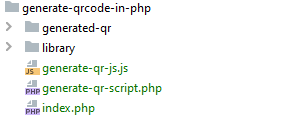
Now its time to create these files, so what are you waiting for ? Let’s start
index.php
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.bundle.min.js"></script> <title>Send Email Example</title> <style> .center-block { display: block; margin-left: auto; margin-right: auto; } </style> </head> <body> <div class="container"> <div class="row"> <div class="col-sm-9 col-md-7 col-lg-5 mx-auto"> <div class="card card-signin my-5"> <div class="card-body"> <h5 class="card-title text-center">Generate QR Code</h5> <form id="generateQrForm" class="form-signin"> <div class="form-label-group"> <label for="inputEmail">Text For QR <span style="color: #FF0000">*</span></label> <input type="text" name="qrText" id="qrText" class="form-control" required placeholder="Enter something to generate QR"> </div> <br/> <div id="generatedQr text-center"> <img src="" id="generatedQrImg" class="center-block"> </div> <br/> <button type="submit" name="generateQrBtn" id="generateQrBtn" class="btn btn-lg btn-primary btn-block text-uppercase" >Generate QR</button> </form> </div> </div> </div> </div> </div> <script type="text/javascript" src="generate-qr-js.js"></script> </body> </html>
generate-qr-script.php
<?php include "library/qrlib.php"; if (isset($_POST['generateQr']) == 'generateQr') { $qrText = $_POST['qrText']; // receive the text for QR $directory = "generated-qr/"; // folder where to store QR $fileName = 'QR-'.rand().'.png'; // generate random name of QR image QRcode::png($qrText, $directory.$fileName, 'L', 4, 2); // library function to generate QR echo "success^".$directory.$fileName; // returns the qr-image path }
generate-qr-js.js
$("form#generateQrForm").on("submit",function (e) { e.preventDefault(); var qrText = $("#qrText").val(); $.post("generate-qr-script.php",{generateQr:'generateQr',qrText:qrText},function (response) { var data = response.split('^'); var generateQrImgPath = data[1]; $("#generatedQrImg").attr("src",generateQrImgPath); }) });
PHP QR code library
Now you need to download the library. it is available in SourceForge. Download it from here.
Output you see

When you enter some text and hit generate QR button, you will get output like this without page reloading. I used jQuery, AJAX to make it.
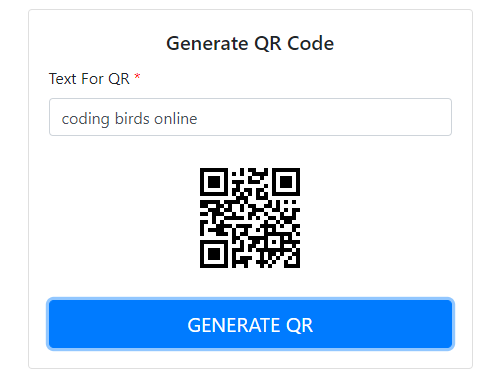
Conclusion
So you learned about how to how to generate QR code with PHP using PHP library and HTML form. If you face any error comment here your problem, and if you want to download the full working source code then you can download it from here.
Happy Coding 🙂