Sending email is mostly used in a variety of websites and web applications. It mostly happens when you send an email by PHP code copied from other websites or blog, it goes to the spam folder. If you want to resolve this issue then you are in the right place. You can check the demo.
Check this also: Send email in PHP using PHPMailer with attachment
mail(to, subject, message, headers, parameters)
This functions 4 required parameters
- to – This is the first parameter required. Put the email id of the person to which you want to send the emails.
- subject – This is the 2nd parameter tells the subject of the mail you want, you can write it.
- message – This is the 3rd parameter. As the name suggests, This is the message what you want to send to recipients.
- headers – This is the 4th parameter which tells about who is sending the email, what is the name of the sender …etc.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="icon" href="https://codingbirdsonline.com/wp-content/uploads/2019/12/cropped-coding-birds-favicon-2-1-192x192.png" type="image/x-icon">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css">
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.slim.min.js"></script>
<script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.bundle.min.js"></script>
<title>Send Email Example</title>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-sm-9 col-md-7 col-lg-5 mx-auto">
<div class="card card-signin my-5">
<div class="card-body">
<h5 class="card-title text-center">Send Email</h5>
<form action="email-script.php" method="post" class="form-signin">
<div class="form-label-group">
<label for="inputEmail">From <span style="color: #FF0000">*</span></label>
<input type="text" name="fromEmail" id="fromEmail" class="form-control" value="info@codingbirdsonline.com" readonly required autofocus>
</div> <br/>
<div class="form-label-group">
<label for="inputEmail">To <span style="color: #FF0000">*</span></label>
<input type="text" name="toEmail" id="toEmail" class="form-control" placeholder="Email address" required autofocus>
</div> <br/>
<label for="inputPassword">Subject <span style="color: #FF0000">*</span></label>
<div class="form-label-group">
<input type="text" id="subject" name="subject" class="form-control" placeholder="Subject" required>
</div><br/>
<label for="inputPassword">Message <span style="color: #FF0000">*</span></label>
<div class="form-label-group">
<textarea id="message" name="message" class="form-control" placeholder="Message" required ></textarea>
</div> <br/>
<button type="submit" name="sendMailBtn" class="btn btn-lg btn-primary btn-block text-uppercase" >Send Email</button>
</form>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
When you will check, you will get this output

In index.php you have <form> tag has the action of email-script.php. This is code for same.
email-script.php
<?php
if (isset($_POST['sendMailBtn'])) {
$fromEmail = $_POST['fromEmail'];
$toEmail = $_POST['toEmail'];
$subjectName = $_POST['subject'];
$message = $_POST['message'];
$to = $toEmail;
$subject = $subjectName;
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
$headers .= 'From: '.$fromEmail.'<'.$fromEmail.'>' . "\r\n".'Reply-To: '.$fromEmail."\r\n" . 'X-Mailer: PHP/' . phpversion();
$message = '<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<span class="preheader" style="color: transparent; display: none; height: 0; max-height: 0; max-width: 0; opacity: 0; overflow: hidden; mso-hide: all; visibility: hidden; width: 0;">'.$message.'</span>
<div class="container">
'.$message.'<br/>
Regards<br/>
'.$fromEmail.'
</div>
</body>
</html>';
$result = @mail($to, $subject, $message, $headers);
echo '<script>alert("Email sent successfully !")</script>';
echo '<script>window.location.href="index.php";</script>';
}
Testing
Now upload to these files to your live server and fill the form, hit the submit button.

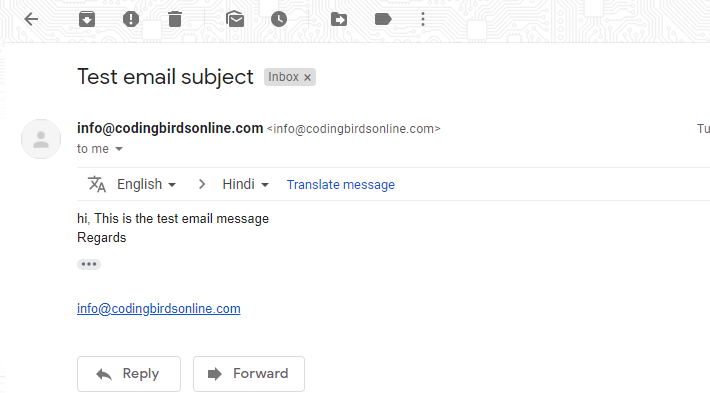
You just need to copy and paste the code nothing else. The code is very simple. And if you still face any problem you can download the source code from here.
You can also watch this video explained the same as in the above post.
Happy coding 🙂